I have made progress in calculating the amount field to respective parent levels in treeDataGrid. But as you see, the original child data became 0 though all parent levels calculation is working well.
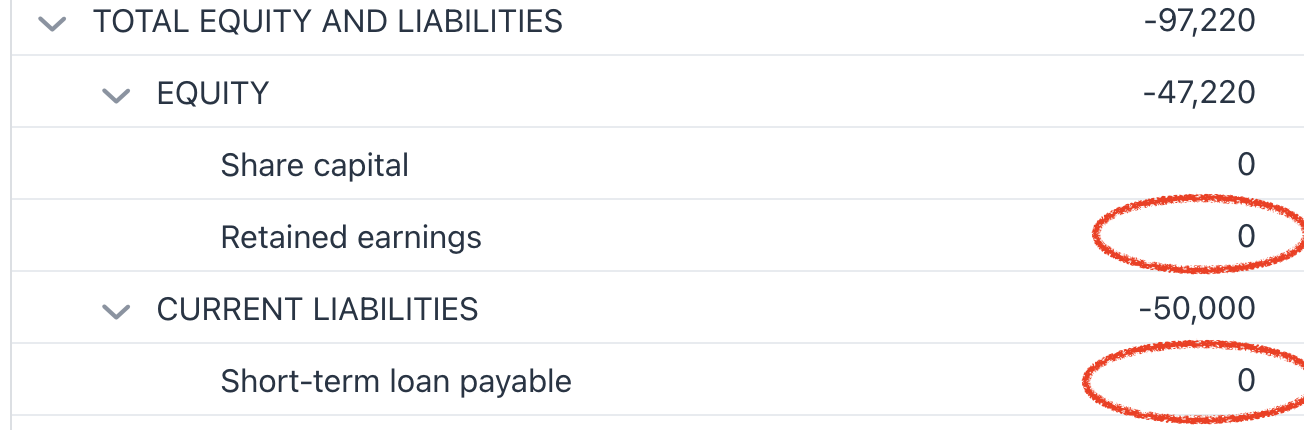
Here is my code, any suggestions to fix this problem to get it working?
private void calcTreeGrid(){
// Create a map to store the child items for each parent item
Map<BalanceSheet, List<BalanceSheet>> childItemsMap = new HashMap<>();
// Create a map to store the sum for each parent item
Map<BalanceSheet, Double> sumMap = new HashMap<>();
// Iterate over each parent row
balanceSheetDc.getItems().forEach(parentItem -> {
double sum = 0.0;
// Retrieve child rows for the current parent row
List<BalanceSheet> childItems = balanceSheetDc.getMutableItems().stream()
.filter(childItem -> Objects.equals(childItem.getParentHead(), parentItem))
.collect(Collectors.toList());
// Calculate the sum using the desired column(s) values
sum = childItems.stream().mapToDouble(BalanceSheet::getAmountCy).sum();
// Set the sum value on the parent row
parentItem.setAmountCy(sum);
// Update the sum in the map
sumMap.put(parentItem, sum);
});
// Restore the original child item values
childItemsMap.forEach((parentItem, childItems) -> {
balanceSheetDc.getMutableItems().removeAll(childItems);
balanceSheetDc.getMutableItems().addAll(childItems);
});
}
The alternative approach as follows but this second approach is also missing the value of original children value like the first approach:
private void calcTotalInTreeGrid(){
// Iterate over each parent row
balanceSheetDc.getItems().forEach(parentItem -> {
final MutableDouble sum = new MutableDouble(0.0);
// Retrieve child rows for the current parent row
balanceSheetDc.getMutableItems().stream()
.filter(childItem -> Objects.equals(childItem.getParentHead(), parentItem))
.forEach(childItem -> {
// Calculate the sum using the desired column(s) values
double value = childItem.getAmountCy();
sum.add(value);
});
// Set the sum value on the parent row
parentItem.setAmountCy(sum.doubleValue());
});
}
public class MutableDouble {
private double value;
public MutableDouble(double value) {
this.value = value;
}
public void add(double value) {
this.value += value;
}
public double doubleValue() {
return value;
}
}