Hi all,
Here is my code look like Dmitry Cherkasov suggestion, but no need remove all column.
@Subscribe
public void onInit(final InitEvent event) {
//remove column by key
if(assetsDataGrid.getColumnByKey("name") !=null) {
assetsDataGrid.removeColumn(assetsDataGrid.getColumnByKey("name"));
}
//Add hierarchy column
TreeDataGrid.Column<Asset> nameColumn= treeDataGrid.addComponentHierarchyColumn(asset -> {
Icon icon ;
if (Boolean.TRUE.equals(asset.getIsFolder())){
icon = ComponentUtils.parseIcon("vaadin:folder");
icon.setColor("#ddd009");
}else {
icon = ComponentUtils.parseIcon("vaadin:file");
icon.setColor("#8383f7");
}
icon.setSize("0.9em");
Span name = new Span(asset.getName());
HorizontalLayout row = new HorizontalLayout(icon, name);
row.setAlignItems(FlexComponent.Alignment.CENTER);
row.setSpacing(true);
return row;
}).setHeader("Name")
.setAutoWidth(true)
.setResizable(true);
//set position for hierarchy column
treeDataGrid.setColumnPosition(nameColumn,0);
}
The result:
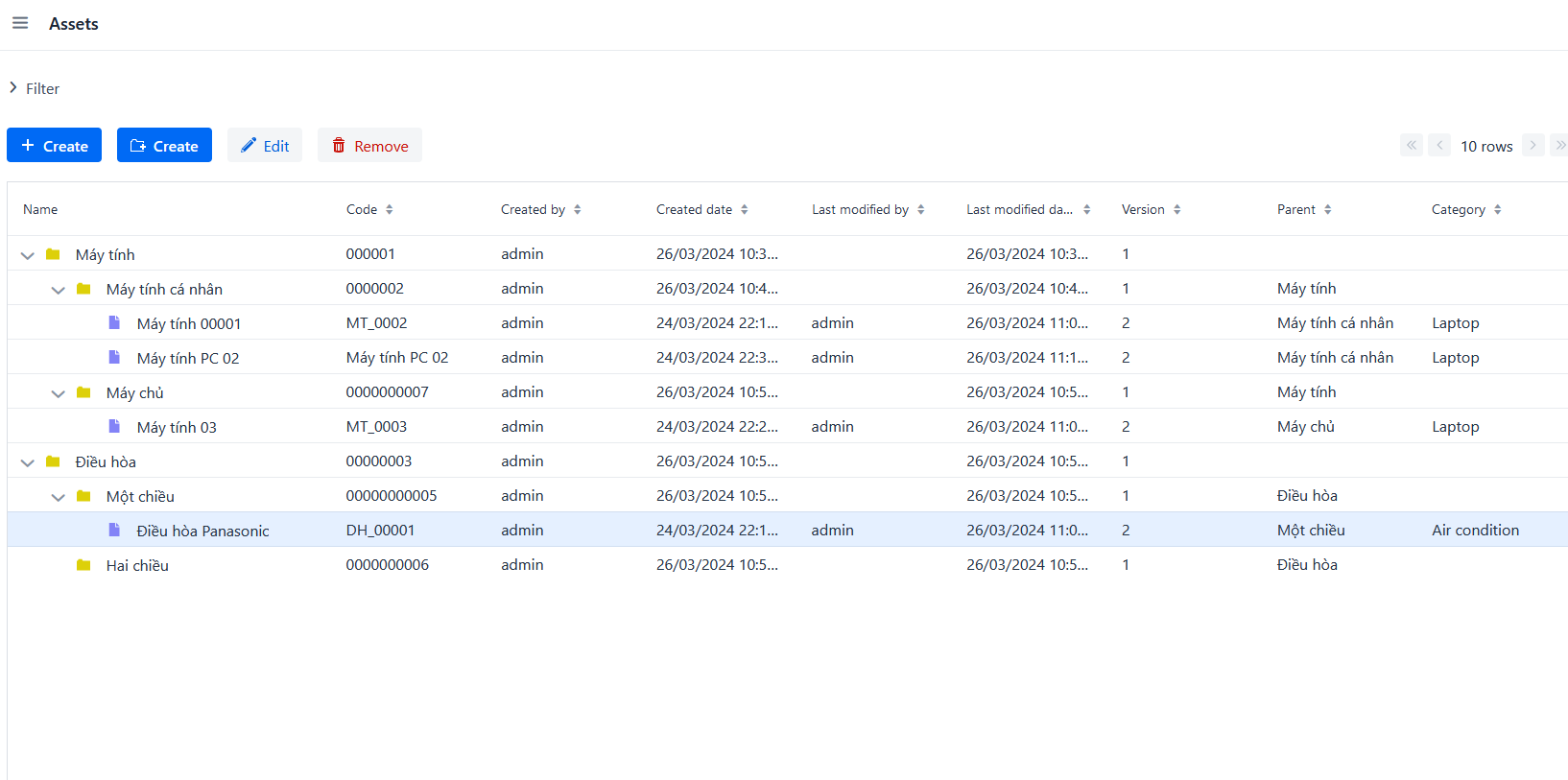